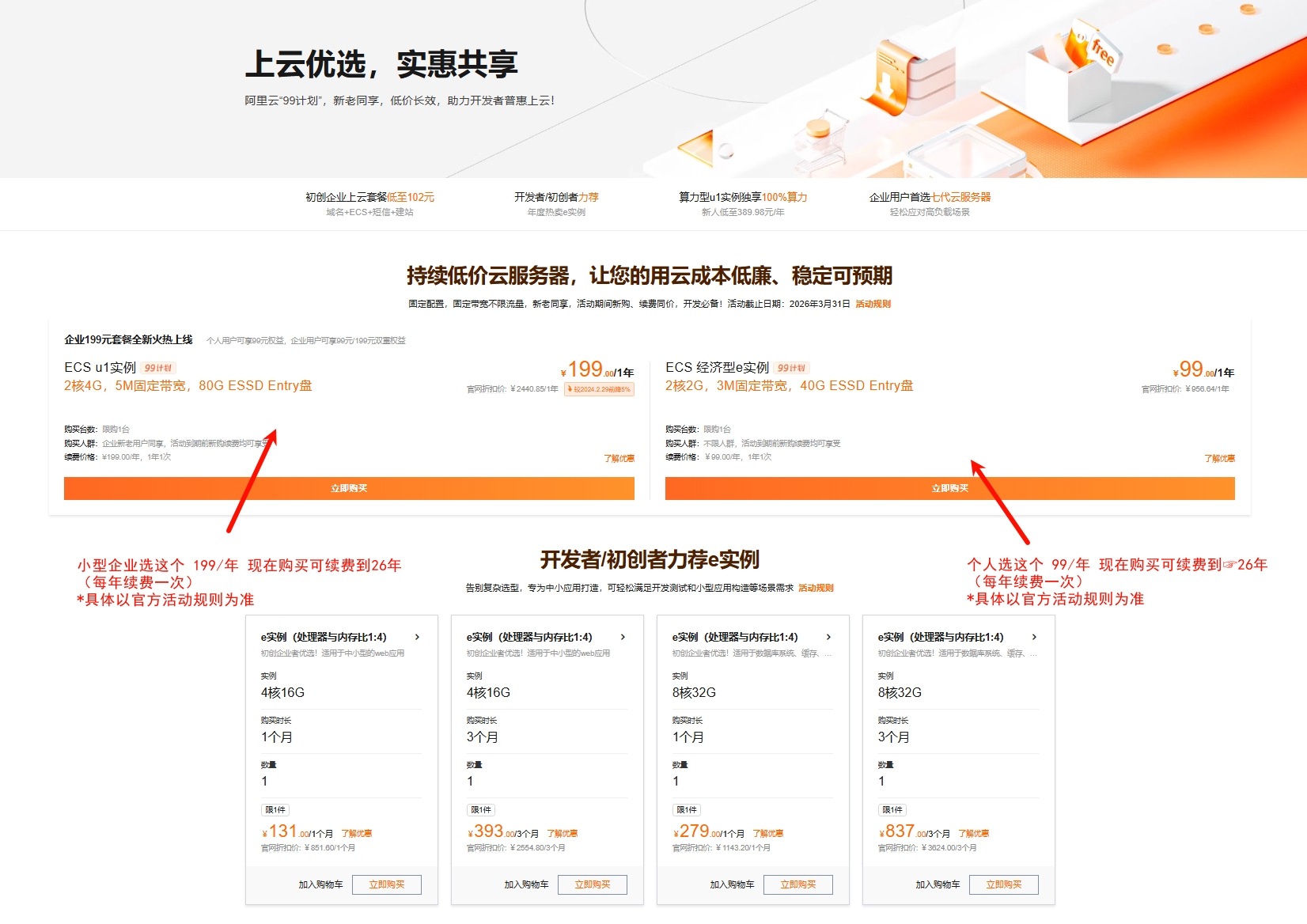
public class ArrayList<E> extends AbstractList<E> implements List<E>, RandomAccess, Cloneable, java.io.Serializable{}
ArrayList<E>类继承了AbstractList<E>抽象类, 实现了List<E>接口, RandomAccess接口, Cloneable接口, java.io.Serializable接口.
private static final long serialVersionUID = 8683452581122892189L;
private static final int DEFAULT_CAPACITY = 10;
↑ DEFAULT_CAPACITY静态常量的作用是 保证ArrayList类默认容量 也就是 10.
private static final Object[] EMPTY_ELEMENTDATA = {};
↑ 当调用构造方法参数为0时,默认设置个空数组.
private static final Object[] DEFAULTCAPACITY_EMPTY_ELEMENTDATA = {};
↑ 当调用无参构造方法时, 默认设置空数组.(和使用0作为参数的返回空数组对象不同)
transient Object[] elementData;
↑ 此数组引用为真正保存数据的引用. transient关键字,用来表示一个域不是该对象串行化的一部分.当一个对象被串行化的时候,transient型变量的值不包括在串行化的表示中,然而非transient型的变量是被包括进去的.
private int size;
↑ size变量用于保存ArrayList对象中的实际元素个数.
private static final int MAX_ARRAY_SIZE = Integer.MAX_VALUE - 8;
↑ 设置数组的最大容量..
public ArrayList(int initialCapacity) { if (initialCapacity > 0) { this.elementData = new Object[initialCapacity]; } else if (initialCapacity == 0) { this.elementData = EMPTY_ELEMENTDATA; } else { throw new IllegalArgumentException("Illegal Capacity: "+ initialCapacity); } }
↑ 构造方法传入默认的capacity. 当initialCapacity大于0时, 设置指定大小的Object数组, 等于0时, 设置一个空数组EMPTY_ELEMENTDATA, 否则抛出IllegalArgumentException异常.
public ArrayList() { this.elementData = DEFAULTCAPACITY_EMPTY_ELEMENTDATA; }
↑ 无参构造方法, 默认设置一个Object空数组 DEFAULTCAPACITY_EMPTY_ELEMENTDATA.
public ArrayList(Collection<? extends E> c) { elementData = c.toArray(); if ((size = elementData.length) != 0) { if (elementData.getClass() != Object[].class) elementData = Arrays.copyOf(elementData, size, Object[].class); } else { this.elementData = EMPTY_ELEMENTDATA; } }
↑ 参数为一个Collection对象的构造方法. 将Collection对象里面的值copy到ArrayList对象中, 若Collection对象中没有值,那么ArrayList对象设置一个空数组.
public boolean add(E e) { ensureCapacityInternal(size + 1); elementData[size++] = e; return true; }
↑ add方法向ArrayList对象末尾中添加数据.每添加一个数据 size的值加1.
public void add(int index, E element) { rangeCheckForAdd(index); ensureCapacityInternal(size + 1); System.arraycopy(elementData, index, elementData, index + 1,size - index); elementData[index] = element; size++; }
↑ add方法向ArrayList对象中指定索引添加数据.每添加一个数据 size的值加1.
private void rangeCheckForAdd(int index) { if (index > size || index < 0) throw new IndexOutOfBoundsException(outOfBoundsMsg(index)); }
↑ rangeCheckForAdd()方法用于判断索引是否越界.如果越界则抛出IndexOutOfBoundsException异常
private void ensureCapacityInternal(int minCapacity) { if (elementData == DEFAULTCAPACITY_EMPTY_ELEMENTDATA) { minCapacity = Math.max(DEFAULT_CAPACITY, minCapacity); } ensureExplicitCapacity(minCapacity); } private void ensureExplicitCapacity(int minCapacity) { modCount++; if (minCapacity - elementData.length > 0) grow(minCapacity); } private void grow(int minCapacity) { int oldCapacity = elementData.length; int newCapacity = oldCapacity + (oldCapacity >> 1); if (newCapacity - minCapacity < 0) newCapacity = minCapacity; if (newCapacity - MAX_ARRAY_SIZE > 0) newCapacity = hugeCapacity(minCapacity); elementData = Arrays.copyOf(elementData, newCapacity); }
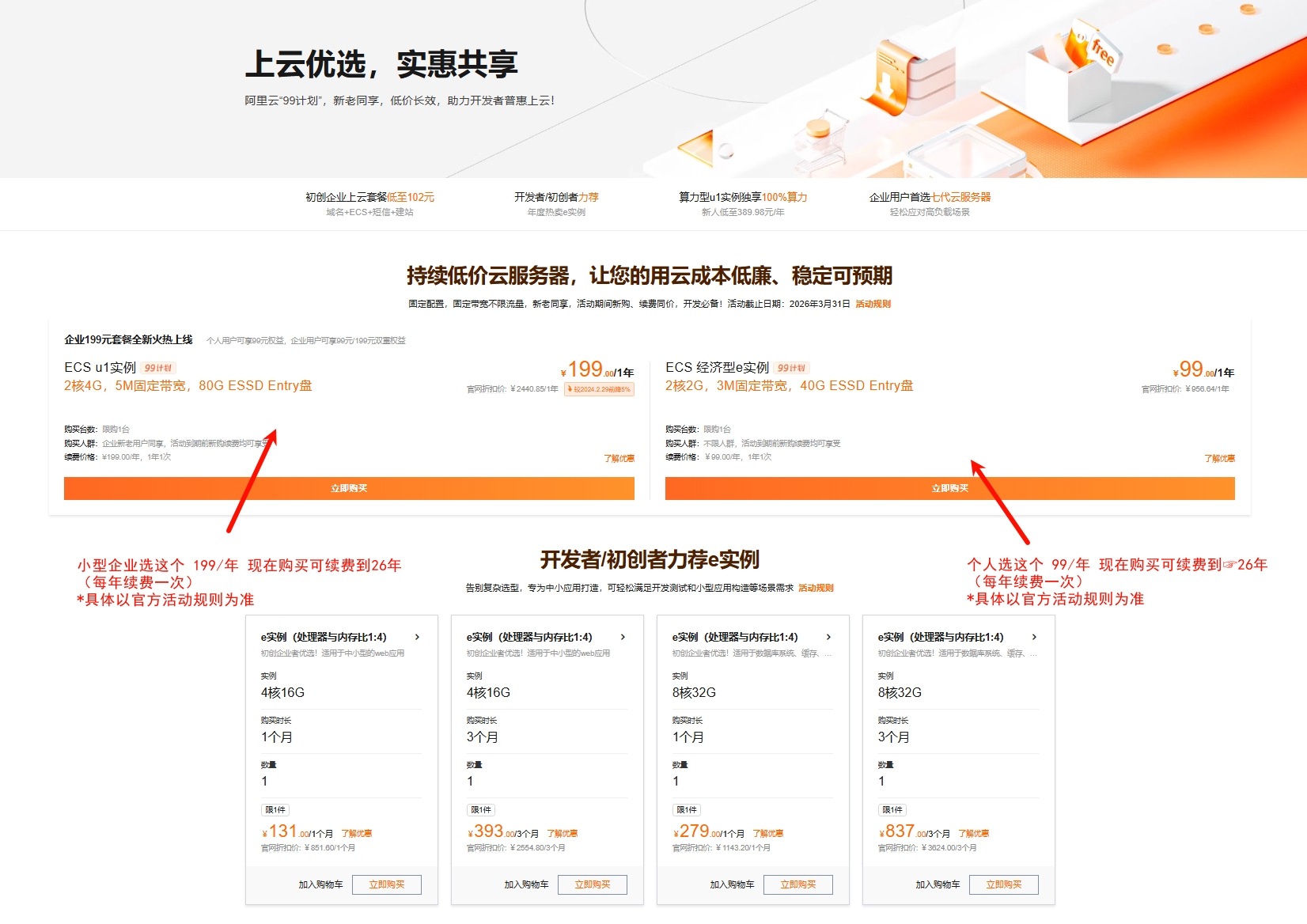